Error call to a member function getcollectionparentid() on null
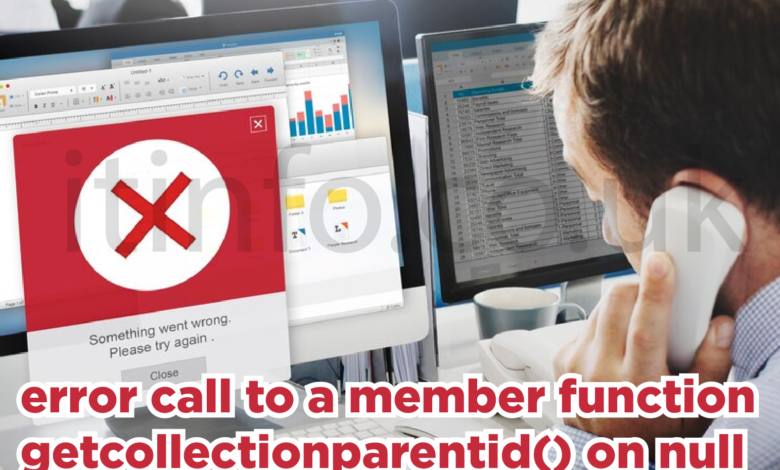
When working with programming languages like PHP, developers frequently encounter various errors related to accessing functions or methods. One such error getcollectionparentid() that can confuse both novice and seasoned developers is:
What Does the Error Mean?
The error message “Call to a member function getCollectionParentId() on null” essentially means that your code is trying to call a method (getCollectionParentId()
) on an object that is null
. This is a common issue in object-oriented programming when an object isn’t properly instantiated or initialized before you attempt to use its methods or properties.
Breakdown of the Error
Let’s break down the error into its components to understand it better:
Call to a member function: This indicates that your code is trying to call a method that belongs to an object. In this case, the method is getCollectionParentId()
.
getCollectionParentId(): This is the method that the code is attempting to call. The function could be part of a class or an object used in your application, often related to collections or hierarchies.
on null: The term “on null” means that the object you’re calling the method on is null
. In other words, it does not exist or has not been initialized properly at the point where you’re trying to access it.
Common Causes of This Error
Several scenarios can lead to this error in PHP applications:
Uninitialized Object: If an object is not properly initialized or instantiated, it remains null
. Trying to call a method on this null
object will result in this error.
Database Query Failures: If your object is supposed to be created or fetched based on a database query and that query fails or returns no results, the object remains null
.
Incorrect Data Flow: Sometimes, the logic flow of your application might not be correctly managed, leading to a situation where a null
object is passed around and eventually has a method called on it.
Typographical Errors: A typo in a variable name or an incorrect object reference could also lead to trying to call a method on null
.
Example of the Error
Here’s a simple example that might result in this error:
$collection = getCollectionById($collectionId); // Suppose this function should return a collection object
// The error occurs here if $collection is null
$parentId = $collection->getCollectionParentId();
In the above code snippet, if getCollectionById($collectionId)
fails or returns null
, then $collection
will be null
. When you try to call getCollectionParentId()
on $collection
, the error is thrown.
How to Fix the Error
To fix this error, consider the following steps:
Check if the Object is Null Before Calling a Method Always ensure that the object is not null
before you attempt to call any methods on it:
$collection = getCollectionById($collectionId);
if ($collection !== null) {
$parentId = $collection->getCollectionParentId();
} else {
// Handle the null case: throw an exception, log an error, or provide a default action
echo "Collection not found!";
}
Ensure Proper Object Initialization Ensure that your object is initialized correctly before you use it. For example, if you’re expecting an object from a function, make sure that function does not return null
.
Verify Database Queries If the object creation or retrieval depends on a database query, make sure that the query is correct and that the database connection is properly established. Also, handle cases where the query might return an empty result set.
Debugging and Logging Use debugging tools or insert logging statements to understand why an object is null
. This will help you trace back to the root cause and fix the logic or flow error.
$collection = getCollectionById($collectionId);
if ($collection === null) {
error_log("Collection with ID {$collectionId} not found.");
}
Exception Handling Implement exception handling mechanisms to manage unexpected null
cases:
try {
$collection = getCollectionById($collectionId);
if ($collection === null) {
throw new Exception("Collection not found");
}
$parentId = $collection->getCollectionParentId();
} catch (Exception $e) {
echo $e->getMessage();
}
Types of Errors When Calling Methods on Null
Call to a Member Function on Null
- Error Message:
Call to a member function methodName() on null
- Description: This is a general error that occurs when you try to call any method (
methodName()
) on a variable that isnull
. - Cause: The variable representing an object was expected to be an instance of a class, but it was actually
null
. - Example:
php $user = getUserById($userId); // Suppose this should return a user object $name = $user->getName(); // Error if $user is null
Trying to Access Property of Non-Object
- Error Message:
Trying to get property 'propertyName' of non-object
- Description: This error occurs when trying to access a property (
propertyName
) on something that is not an object. It often happens when an object is expected butnull
is encountered instead. - Cause: The variable holding an object is
null
or is not an object at all. - Example:
php $user = getUserById($userId); // Suppose this should return a user object echo $user->name; // Error if $user is null
Call to a Member Function on Boolean
- Error Message:
Call to a member function methodName() on boolean
- Description: This error happens when a method is called on a variable that is a boolean (
true
orfalse
) instead of an object. - Cause: The code is expecting an object but receives a boolean, often due to incorrect handling of function return values (e.g., a function that returns
false
on failure). - Example:
php $file = fopen('nonexistentfile.txt', 'r'); // This returns false if the file does not exist $file->read(); // Error if $file is false
- Also Read: https://fashionviko.com
Member Function on Undefined Variable
- Error Message:
Call to a member function methodName() on undefined variable
- Description: This occurs when attempting to call a method on a variable that hasn’t been defined.
- Cause: A variable is being used before it is declared or initialized.
- Example:
php echo $undefinedVar->someMethod(); // Error because $undefinedVar is not defined
Call to a Member Function on Array
- Error Message:
Call to a member function methodName() on array
- Description: This happens when you try to call an object method on an array.
- Cause: A misunderstanding or typo in code logic might lead to an array being mistaken for an object.
- Example:
php $data = ['name' => 'Alice', 'age' => 30]; echo $data->getName(); // Error because $data is an array, not an object
Undefined Method Error
- Error Message:
Call to undefined method ClassName::methodName()
- Description: This error occurs when a method is called on an object, but the method does not exist in the object’s class.
- Cause: Typo in the method name, or the method has not been defined in the class.
- Example:
class User { public function getName() { return 'John Doe'; } } $user = new User(); echo $user->getFullName(); // Error because getFullName() does not exist in the User class
How to Handle These Errors
Null Checks Before Method Calls: Always check if the variable is not null
before calling a method on it.
Verify Return Types: Make sure that functions return the expected types and handle different cases (like false
or null
).
Initialize Variables Properly: Ensure all variables are initialized before using them.
Exception Handling: Use try-catch blocks to handle exceptions gracefully, providing error messages or fallbacks when something unexpected happens.
Debugging and Logging: Use logging to understand where your code is failing and debug more effectively.
Type Hinting and Validation: Utilize PHP’s type hinting and validations to ensure functions receive and return the correct types of data getCollectionParentId().
Conclusion
Handling errors getCollectionParentId() effectively is a crucial skill in software development. Understanding the types of errors that can occur when calling methods on null
or incorrect types helps you write more robust and error-resistant code. By using proper checks, validation, and debugging techniques, you can avoid these errors and build more reliable applications.
Also Read This: Fix the Error: errordomain=nscocoaerrordomain&errormessage=could not find the specified shortcut.&errorcode=4